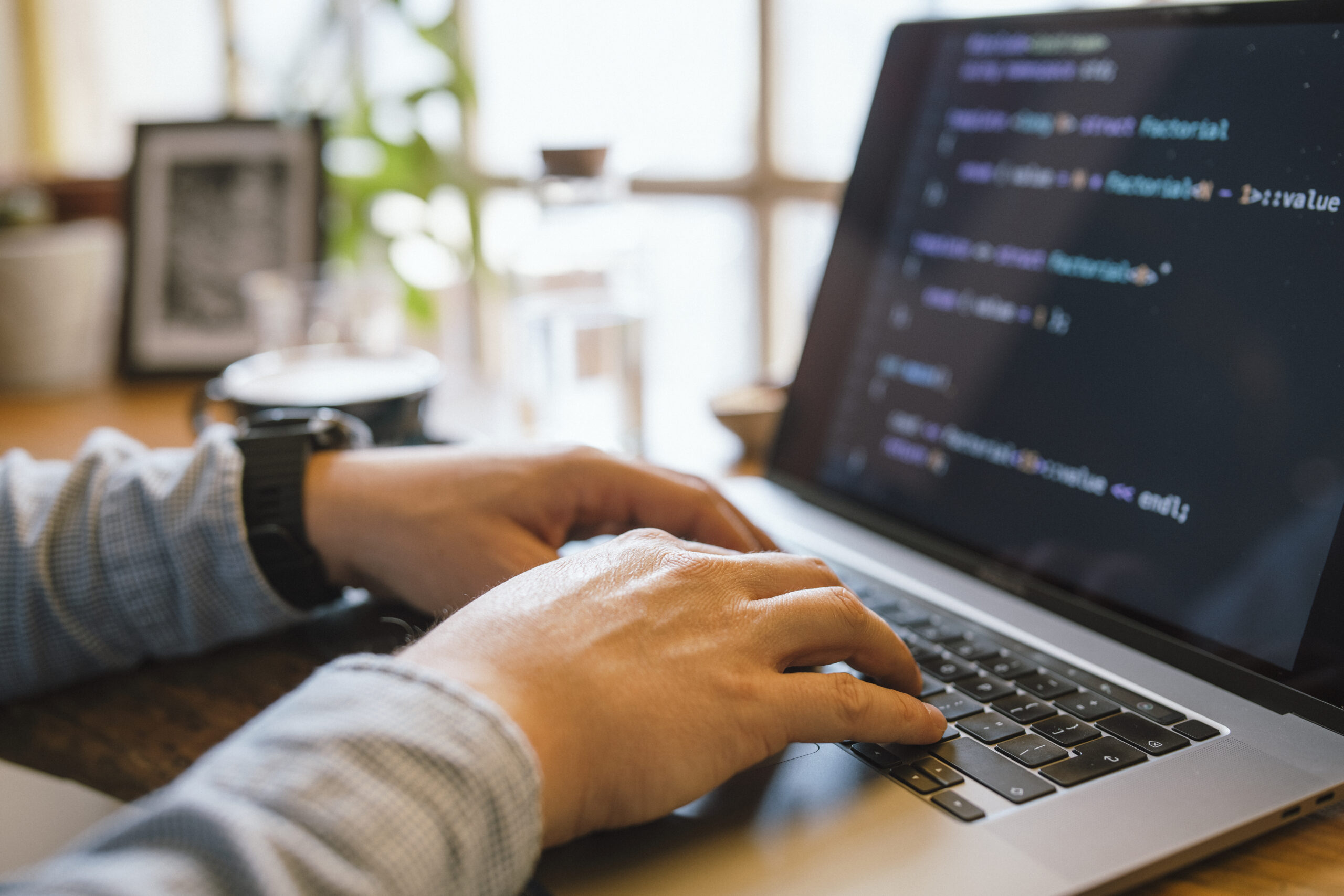
Debugging is one of the most crucial — nonetheless often ignored — capabilities in the developer’s toolkit. It isn't almost correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Mastering to Assume methodically to unravel complications competently. Whether you are a newbie or perhaps a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productiveness. Listed below are numerous methods to assist developers amount up their debugging match by me, Gustavo Woltmann.
Master Your Tools
One of many fastest techniques builders can elevate their debugging competencies is by mastering the instruments they use daily. Whilst writing code is one Element of advancement, realizing the best way to interact with it correctly through execution is equally essential. Fashionable development environments occur Outfitted with powerful debugging abilities — but several builders only scratch the floor of what these equipment can do.
Acquire, by way of example, an Integrated Progress Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step by code line by line, and even modify code about the fly. When utilized the right way, they Allow you to notice precisely how your code behaves all through execution, which happens to be invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for entrance-conclude developers. They help you inspect the DOM, keep track of community requests, view actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become comfy with Edition Management units like Git to understand code heritage, obtain the exact moment bugs had been introduced, and isolate problematic alterations.
Finally, mastering your resources signifies heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your advancement surroundings to ensure when difficulties occur, you’re not missing in the dead of night. The greater you know your tools, the more time you can spend resolving the particular problem rather than fumbling through the procedure.
Reproduce the condition
One of the more significant — and infrequently ignored — actions in effective debugging is reproducing the problem. Right before leaping to the code or producing guesses, builders want to create a consistent ecosystem or circumstance in which the bug reliably appears. With out reproducibility, fixing a bug will become a match of likelihood, often bringing about wasted time and fragile code adjustments.
The first step in reproducing a dilemma is collecting just as much context as is possible. Request concerns like: What actions triggered The problem? Which environment was it in — enhancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you have got, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
As you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood environment. This might suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, take into account writing automated checks that replicate the edge circumstances or point out transitions involved. These exams not just support expose the problem but in addition protect against regressions in the future.
Often, The difficulty could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than certain configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating such bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, in addition to a methodical approach. But when you can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible situation, You need to use your debugging tools more successfully, check prospective fixes securely, and talk much more clearly using your staff or end users. It turns an summary grievance into a concrete challenge — and that’s where developers thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when a little something goes Erroneous. In lieu of observing them as annoying interruptions, developers ought to learn to take care of mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the information thoroughly and in total. Numerous builders, particularly when below time tension, glance at the very first line and straight away get started building assumptions. But deeper during the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste mistake messages into engines like google — read and fully grasp them very first.
Crack the error down into pieces. Is it a syntax mistake, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate triggered it? These inquiries can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can substantially increase your debugging procedure.
Some glitches are vague or generic, As well as in Those people instances, it’s critical to look at the context by which the error transpired. Look at relevant log entries, enter values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings either. These usually precede much larger concerns and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong equipment in a developer’s debugging toolkit. When used successfully, it provides real-time insights into how an application behaves, helping you comprehend what’s happening beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method starts with realizing what to log and at what degree. Typical logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts through growth, Facts for normal functions (like productive begin-ups), Alert for opportunity difficulties that don’t split the application, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with excessive or irrelevant details. Too much logging can obscure vital messages and slow down your method. Deal with critical functions, state improvements, input/output values, and important decision factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-believed-out logging technique, you can lessen the time it takes to spot difficulties, gain deeper visibility into your programs, and Increase the overall maintainability and reliability of the code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently detect and repair bugs, developers will have to approach the process just like a detective fixing a thriller. This way of thinking allows break down complicated concerns into workable pieces and follow clues logically to uncover the root trigger.
Begin by collecting evidence. Consider the indicators of the situation: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather as much related info as you are able to without having leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to your self: What might be causing this actions? Have any variations not long ago been designed on the codebase? Has this concern occurred before below similar instances? The purpose is always to narrow down choices and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside a managed setting. In the event you suspect a selected purpose or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, check with your code issues and Allow the effects direct you closer to the reality.
Pay shut interest to compact information. Bugs frequently hide from the least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may perhaps conceal the actual problem, only for it to resurface afterwards.
And finally, continue to keep notes on Everything you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others realize your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Produce Checks
Writing exams is one of the best solutions to help your debugging abilities and All round growth effectiveness. Assessments not just aid catch bugs early but in addition function a security Web that offers you assurance when making changes for your codebase. A nicely-analyzed software is simpler to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which target unique capabilities or modules. These compact, isolated checks can promptly expose no matter whether a certain piece of logic is Operating as expected. When a exam fails, you instantly know exactly where to look, significantly lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear right after previously getting fixed.
Future, combine integration exams and finish-to-end assessments into your workflow. These aid make sure that various portions of your application perform together effortlessly. They’re specifically helpful for catching bugs that manifest in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Consider critically about your code. To test a feature appropriately, you need to be aware of its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way potential customers to higher code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug might be a robust first step. As soon as the check fails continually, you are able to target correcting the bug and observe your take a look at go when the issue is settled. This tactic makes certain that exactly the same bug doesn’t return Sooner or later.
In brief, composing checks turns debugging from the irritating guessing recreation right into a structured and predictable system—helping you catch a lot more bugs, speedier and more reliably.
Get Breaks
When debugging a difficult issue, it’s simple to become immersed in the challenge—observing your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Taking breaks helps you reset your mind, decrease disappointment, and sometimes see the issue from a new perspective.
When you're too near the code for much too long, cognitive exhaustion sets in. You may perhaps begin overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A read more brief stroll, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Numerous builders report acquiring the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially throughout longer debugging classes. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Electricity as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you in advance of.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then have a 5–10 moment break. Use that point to maneuver close to, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it in fact contributes to a lot quicker and more effective debugging In the long term.
In short, getting breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and helps you avoid the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It really is a possibility to mature as being a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, each can train you a little something valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots with your workflow or comprehension and allow you to Make more robust coding habits moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. After some time, you’ll begin to see designs—recurring problems or common mistakes—you could proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically potent. Whether it’s via a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals steer clear of the similar concern boosts group efficiency and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important aspects of your advancement journey. After all, several of the best builders are not the ones who generate great code, but those that repeatedly discover from their faults.
In the end, Each individual bug you resolve provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging abilities can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The next time you are knee-deep in a very mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.